Super Chip Emulator
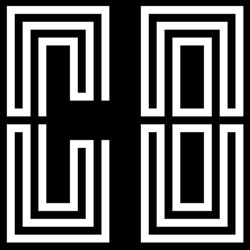
This is a Super Chip and Chip8 emulator done with Python 3.6 and Pygame. This project is divided in several modules for a better organization.
The source code can be acessed here.
Dependencies
This project uses the library pygame, if you don’t have it you can install it with the following command.
pip3 install -r requirements.txt
How to Use
There are two ways to use this emulator, via terminal or user interface.
Terminal:
python3 main.py path/to/rom
User Interface
The path used for search is the ../roms.
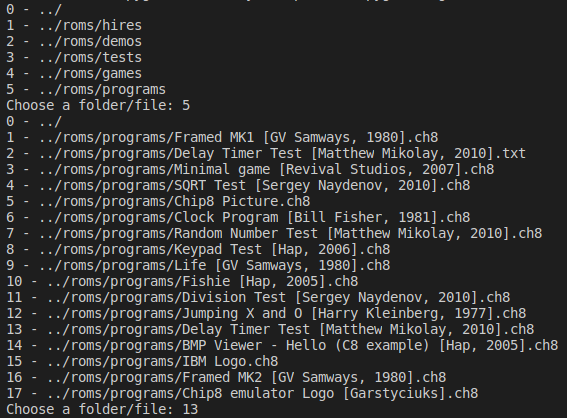
Configuration
You can change a set of configurations like the colors used by the emulator or the keys. You will find more information in the src/config.ini file
Super Chip and Chip 8
The Super Chip has backward compatibility with Chip8, using a flag to change between the two modes.
The Chip8 and Super Chip games range from Pong to Tetris and even a mario clone (Ant).
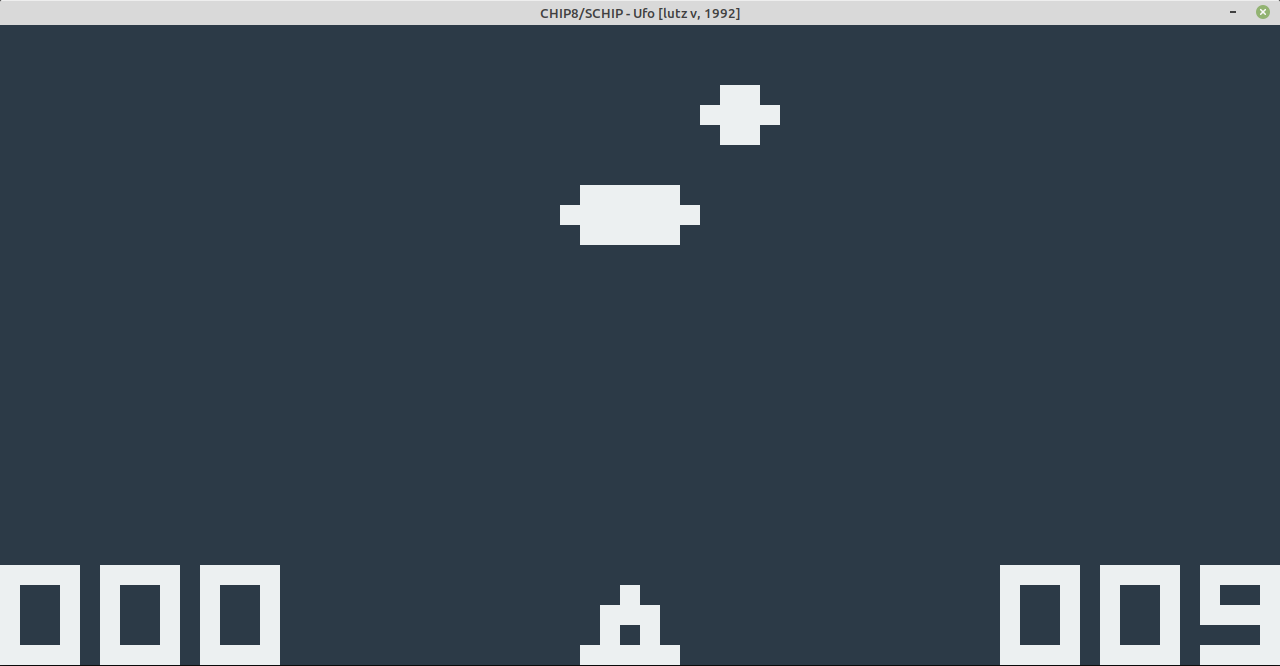
UVO - Chip 8
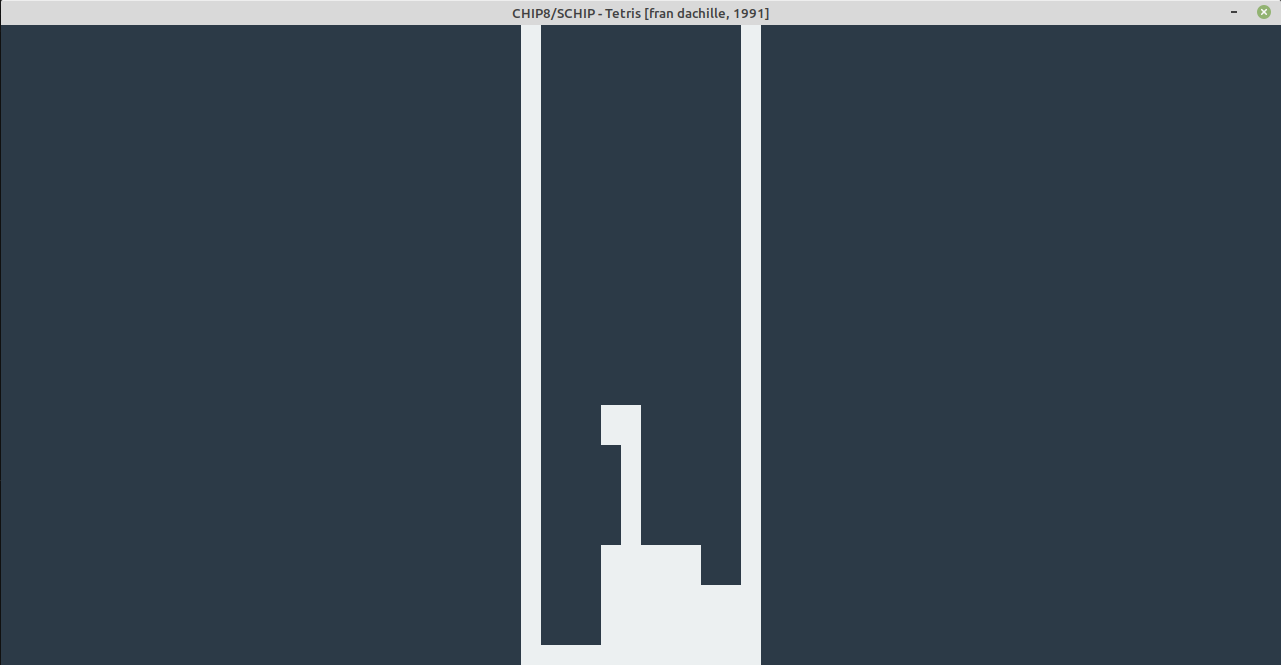
Tetris - Chip 8
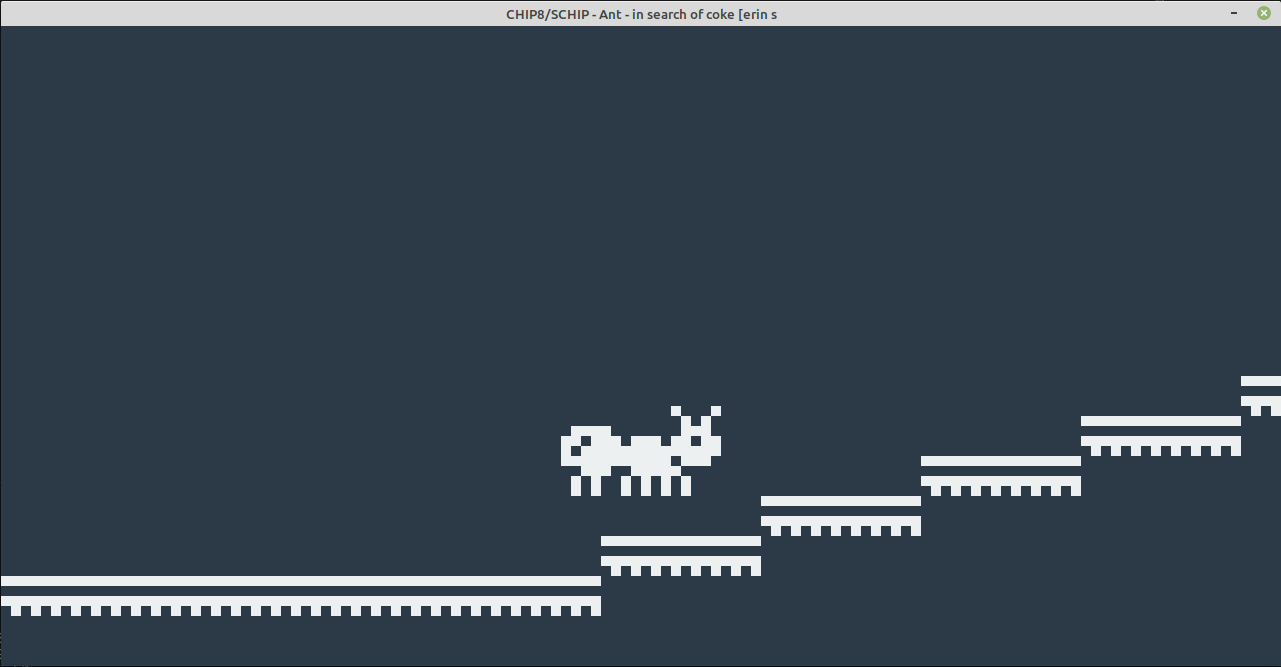
Ant - Super Chip
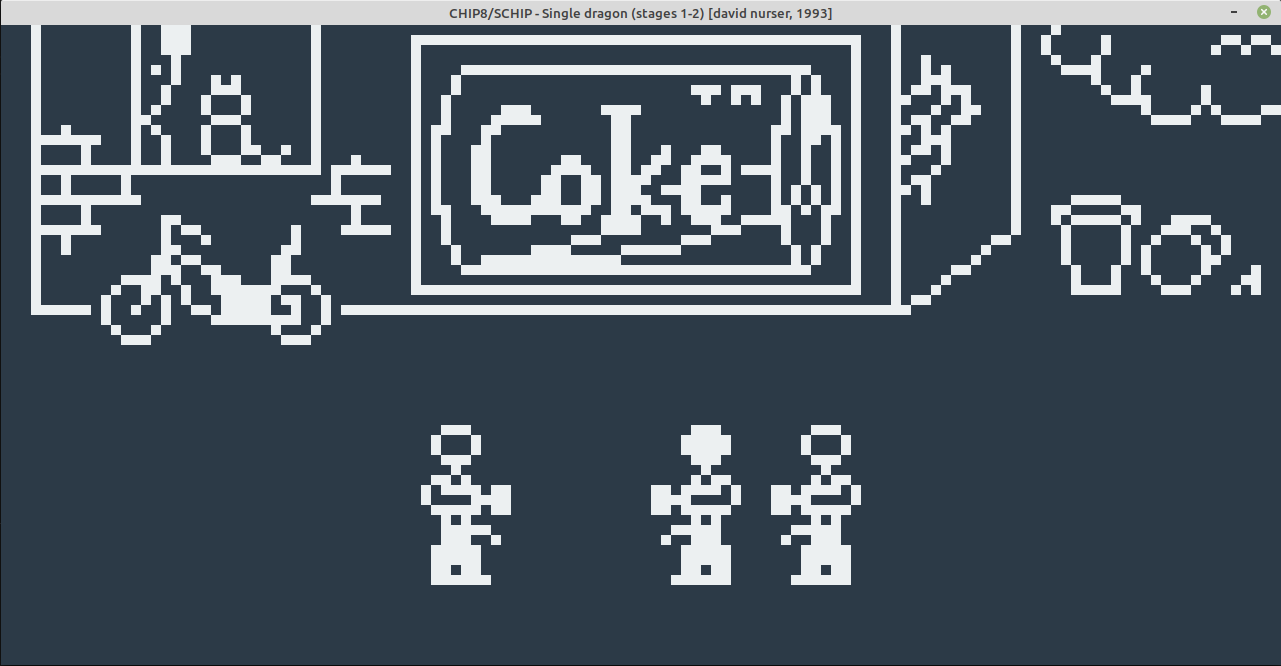
Single Dragon - Super Chip
CPU
The Chip8 CPU disposes of a set of registers and timers:
PC : Program Counter - The current address memory I : The address register Opcode : The opcode that will be executed by the CPU
Delay timer: Used for timing the events of games. Sound timer: Used for sound effects (a beep is played when reaches 0).
CPU Registers
Chip8 as 16 8 bit registers, V0 to V0xF.
V0xF is used for flags (carry, not borrow, etc.).
Along with the registers, there is also a Stack used to keep track of the return locations during a function call.
Opcodes
The opcodes are 16 bit codes executed by the CPU, they range from simple arithmetic operations to loading sprites from the memory.
In Chip8 the last four bits of the opcode (opcode & 0xF000) are used for identification.
Some examples:
Opcode | C Code | Description |
---|---|---|
0x6XNN | V[X] = NN | Sets V[x] to NN |
0x9XY0 | if(V[X] != V[Y]) | Skips the next instruction if V[X] doesn’t equal V[Y] |
0xFX18 | sound_timer = v[X] | Sets the sound timer to V[X] |
Description:
NNN = opcode & 0x0FFF NN = opcode & 0x00FF N = opcode & 0x000F Y = (opcode & 0x00F0) » 4 X = (opcode & 0x0F00) » 8 V = The sixteen CPU registers
The complete official CHip8 opcode list can be accessed in link.
Memory
RAM
The Chip8 has 4KB of memory, 4096 memory locations of 8 bits. The first 512 addresses are reserved to the Chip8 interpreter so the programs start at the location 0x200 (the default value of PC).
+----------------+= 0xFFF End of Chip-8 RAM
| |
| |
| 0x200 to 0xFFF |
| Chip-8 Program |
| |
| |
+----------------+= 0x200 Start of most Chip-8 programs
| Reserved for |
| interpreter |
+----------------+= 0x000 Start of Chip-8 RAM
Diagram based on the Cowgod’s Chip-8 Technical Reference v1.0
Input
The Chip8 input is done via a 16 key keyboard with the hexadecimal codes from 0x0 to 0xF.
The diagram bellow relates the used keys to their hexadimal codes.
+---+---+---+---+ +---+---+---+---+
|0x1|0x2|0x3|0xC| | 1 | 2 | 3 | 4 |
+---+---+---+---+ +---+---+---+---+
|0x4|0x5|0x6|0xD| | Q | W | E | R |
+---+---+---+---+ >> +---+---+---+---+
|0x7|0x8|0x9|0xE| | A | S | D | F |
+---+---+---+---+ +---+---+---+---+
|0xA|0x0|0xB|0xF| | Z | X | C | V |
+---+---+---+---+ +---+---+---+---+
Sound
Chip8 supports only a beep that is played when the sound timer reaches zero.
Display
The Super Chip and Chip 8 have different display resolutions, Chip8 has a 64 by 32 and Super Chip a 128 by 64. In order to keep everything running smoothly the Chip8 is scaled to match the resolution of the Super Chip.
Super Chip has a monochromatic 128 by 64 pixels display.
(0,0) (127, 0)
+---------------------+
| |
| |
| |
+---------------------+
(63, 0) (127, 63)
Super Chip Display
Chip8 has a monochromatic 64 by 32 pixels display.
(0,0) (63, 0)
+---------+
| |
+---------+
(63, 0) (63, 31)
Chip8 Display
References
- http://devernay.free.fr/hacks/chip8/C8TECH10.HTM
- https://en.wikipedia.org/wiki/CHIP-8
- http://devernay.free.fr/hacks/chip8/schip.txt
- https://www.google.pt/search?client=opera&q=super+chip+documentations
- http://www.pong-story.com/chip8/